Table of Contents
Login with Facebook and Google Account on website in PHP
In previous days every website maintains their user records with signup form process and keeping the records in the database. But, now days no need of full fills a sign up form for become a user of website. Now user get directly login instead of signup process with help of social API. Here we are going to introduce Google login with OAuth and Facebook login using SDK.
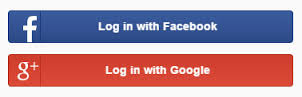
For this process we need Google client ,client secret key and Registered Redirect URI and app id and app secret for facebook.
When we get Facebook and Google details for which discussed above we move to config file and mention required id and secret key.
Config.php file
<?php // PHP Cluster - http://www.phpcluster.com/ session_start(); define('BASE_URL', filter_var('http://localhost/social/', FILTER_SANITIZE_URL)); // to get client id and client secret Visit https://code.google.com/apis/console to generate your oauth2_client_id, oauth2_client_secret, and to register your oauth2_redirect_uri. define('CLIENT_ID','OAUTH CLIENT ID'); define('CLIENT_SECRET','OAUTH CLIENT SECRET'); define('REDIRECT_URI','OAUTH REDIRECT URI');//example:http://localhost/social/login.php?google,http://example/login.php?google define('APPROVAL_PROMPT','auto'); define('ACCESS_TYPE','offline'); //For facebook login define('APP_ID','FACEBOOK APP ID'); define('APP_SECRET','FACEBOOK APP SECRET'); ?>
Here in config.php we are defining access type offline. Google Oauth login provides two types of login access which are Online and Offline.Defining approval promt as auto here.
Let us discuss about index.php file code which is shown below:
Index.php file
<?php // PHP Cluster - http://www.phpcluster.com/ require_once 'config.php'; ?> <!doctype html> <html> <head><meta charset="utf-8"> <title>Login with Social Accounts- PHP Cluster</title> <script src="http://ajax.googleapis.com/ajax/libs/jquery/1.7.1/jquery.min.js" type="text/javascript"></script> <script type="text/javascript" src="js/oauthpopup.js"></script> <script type="text/javascript"> $(document).ready(function(){ //For Google $('a.login').oauthpopup({ path: 'login.php?google', width:550, height:350, }); $('a.google_logout').googlelogout({ redirect_url:'<?php echo $base_url; ?>logout.php?google' }); //For Facebook $('#facebook').oauthpopup({ path: 'login.php?facebook', width:500, height:300, }); }); </script> </head> <body> <div style="float:left;width:33%;margin-left:30%;"> <?php if(!isset($_SESSION['User'])) { ?> <div style="margin-left:10%;float:left;width:300px;"> <img src='images/facebook.png' id='facebook' style='cursor:pointer;float:left;margin-right:10%;' alt="Sign in with Facebook"/> <a class='login' href='javascript:void(0);' style="float:left;"><img alt='Signin in with Google' src='images/google.png' /></a> </div> <?php } else { if(isset($_SESSION['facebook_logout'])){ ?> <img src="https://graph.facebook.com/<?php echo $_SESSION['User']['id']; ?>/picture" width="50"/><div><?php echo $_SESSION['User']['name'];?></div> <a href="<?php echo $_SESSION['facebook_logout'];?>">Logout</a> <?php } else{ ?> <img src="<?php echo $_SESSION['User']['picture']; ?>" width="50" /><div><?php echo $_SESSION['User']['name'];?></div> <div><?php echo $_SESSION['User']['email']; ?></div> <a class='google_logout' href='javascript:void(0);'>Logout</a> <?php } } ?> </div> </body></html>
Here in index.php we are using session for checking user login .In this we are using Jquery for creating popup for google and facebook login using API.
Login.php
<?php // PHP Cluster - http://www.phpcluster.com/ require 'Social.php'; $Social_obj= new Social(); if(isset($_GET['facebook'])){ $Social_obj->facebook(); } if(isset($_GET['google'])){ $Social_obj->google(); } ?> <!-- after authentication popup will close using this script --> <script type="text/javascript"> window.close(); </script>
Social.php
<?php // PHP Cluster - http://www.phpcluster.com/ //For Facebook require_once 'config.php'; require_once 'lib/facebook/facebook.php'; //For Google require_once 'lib/google/Google_Client.php'; require_once 'lib/google/Google_Oauth2Service.php'; class Social{ function facebook(){ $facebook = new Facebook(array( 'appId' => APP_ID, //your app id 'secret' => APP_SECRET, // your secret key )); //get the user facebook id $user = $facebook->getUser(); //echo $user;exit; if($user){ try{ //get the facebook user profile data $user_profile = $facebook->api('/me'); $params = array('next' => BASE_URL.'logout.php'); //logout url $logout =$facebook->getLogoutUrl($params); $_SESSION['User']=$user_profile; $_SESSION['facebook_logout']=$logout; }catch(FacebookApiException $e){ error_log($e); $user = NULL; } } if(empty($user)){ //login url $loginurl = $facebook->getLoginUrl(array( 'scope' => 'email,read_stream, publish_stream, user_birthday, user_location, user_work_history, user_hometown, user_photos', 'redirect_uri' => BASE_URL.'login.php?facebook', 'display'=>'popup' )); header('Location: '.$loginurl); } } function google(){ $client = new Google_Client(); $client->setApplicationName("Google Login Function"); $client->setClientId(CLIENT_ID); $client->setClientSecret(CLIENT_SECRET); $client->setRedirectUri(REDIRECT_URI); $client->setApprovalPrompt(APPROVAL_PROMPT); $client->setAccessType(ACCESS_TYPE); $oauth2 = new Google_Oauth2Service($client); if (isset($_GET['code'])) { $client->authenticate($_GET['code']); $_SESSION['token'] = $client->getAccessToken(); } if (isset($_SESSION['token'])) { $client->setAccessToken($_SESSION['token']); } if (isset($_REQUEST['error'])) { echo '<script type="text/javascript">window.close();</script>'; exit; } if ($client->getAccessToken()) { $user = $oauth2->userinfo->get(); $_SESSION['User']=$user; $_SESSION['token'] = $client->getAccessToken(); } else { $authUrl = $client->createAuthUrl(); header('Location: '.$authUrl); } } } ?>
In this social.php file we have created function for social login access using api id and secret key for google and facebook separately.
Logout.php
<?php // PHP Cluster - http://www.phpcluster.com/ require_once 'config.php'; if($_GET['google']){ require_once 'lib/google/Google_Client.php'; $client = new Google_Client(); unset($_SESSION['token']); $client->revokeToken(); } if(isset($_SESSION['User']) && !empty($_SESSION['User'])){ session_destroy(); } header('Location: '.BASE_URL); ?>
Logout.php file will redirect user to index.php for login again after destroy session of user for facebook and unset token of google for user.