Google OAuth Login allow user to login with their Google account to your website. In our previous post we have learned how to login with Facebook using PHP. In this tutorial we will see how integrate login with Google system in your website. In this tutorial we will see how to authenticate a user to access website with Google Account credentials. For getting Google OAuth permission to authenticate a user we have to follow some steps which are as follows.
• Create a Project in Google API Console and Get all OAuth Credentials.
• Download Google API Lib and upload it to include in your webpage.
There are some steps which have to follow after above project creation process.
• Send Request for authenticate a user with their credentials
• Access user info from Google and save to database if not exist in it.
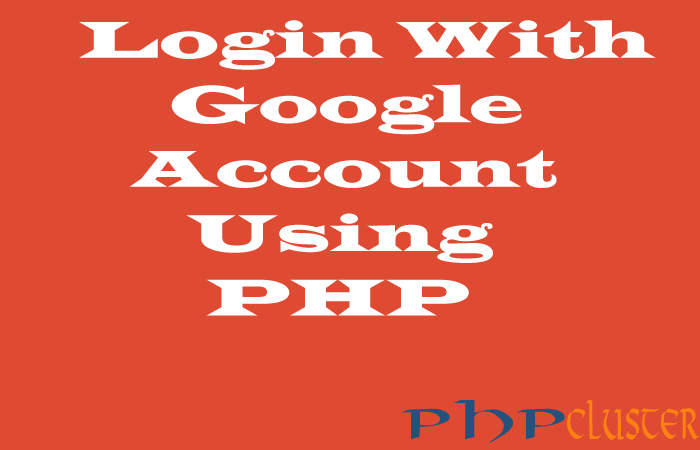
Demo
Let us see snippet in detail:
Step 1: Go to Google Developer Console and Click on Goole + API . Go through this URL https://console.developers.google.com/
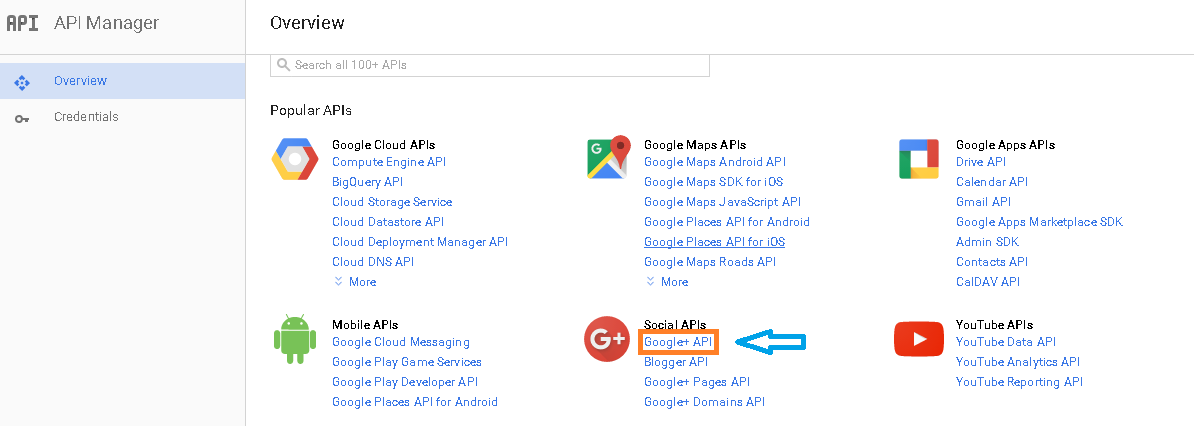
Step 2: Create a new project to get OAuth login detail. Click on Create a project button. For reference see given below picture.
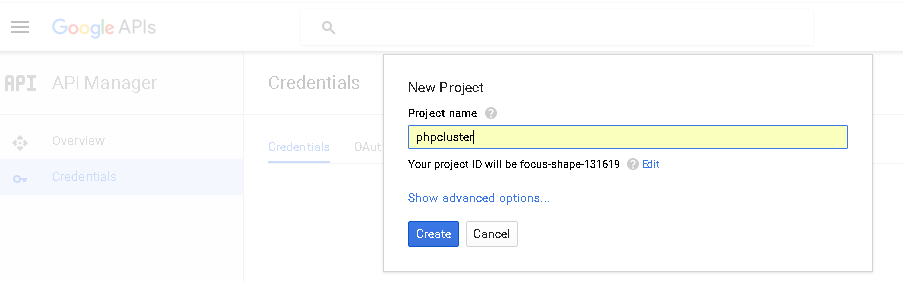
Write Your project name and agree to Terms of service and click on create.
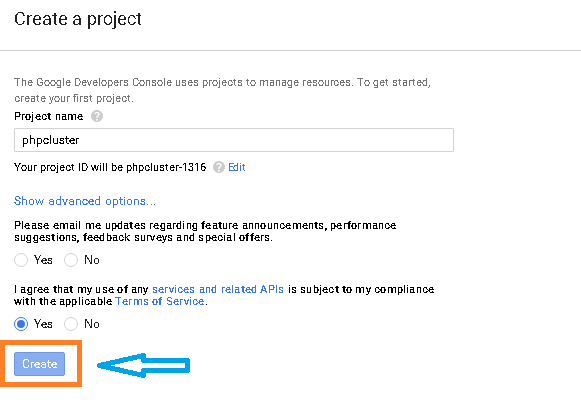
Now Enable API to get work your project created. After enable you get option to go to credential. Click on Go to the credential button
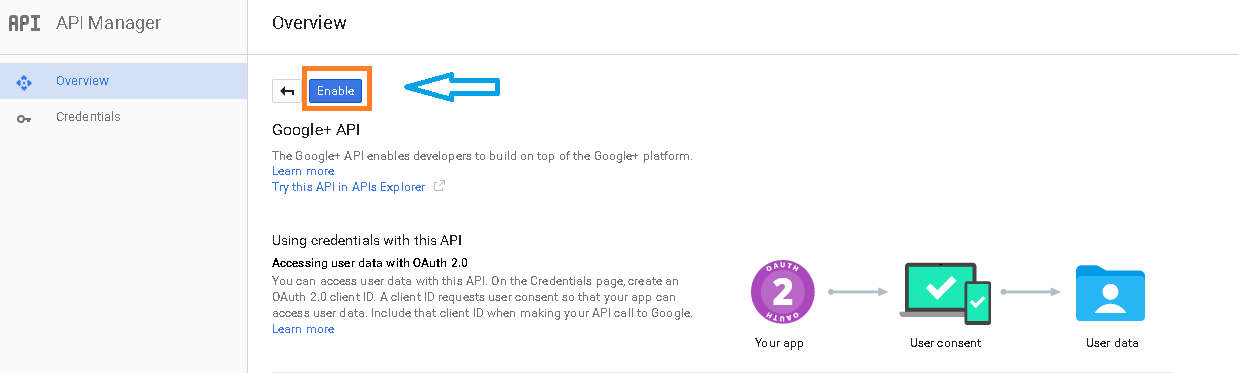
Add credential to your project as shown in below picture. Fill all detail to complete your credentials. Now click on Create Client ID button.
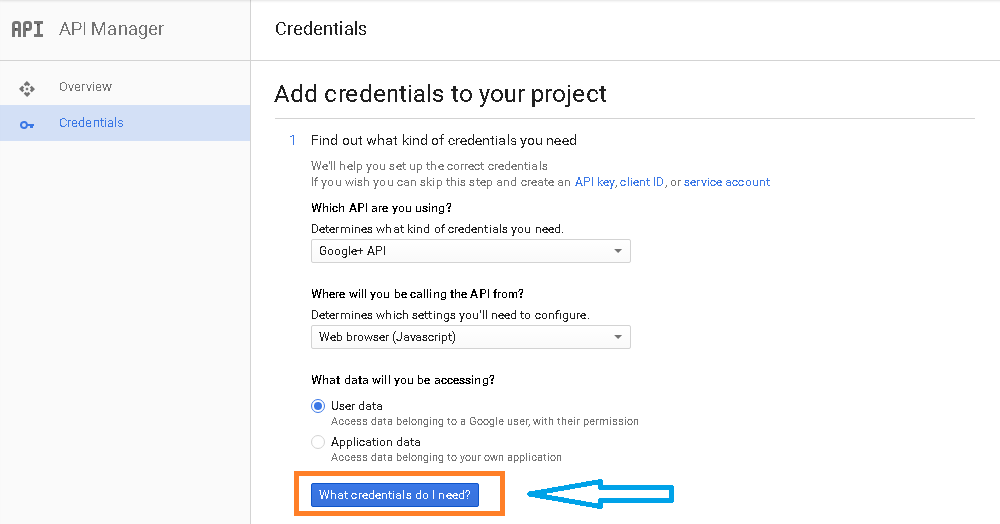
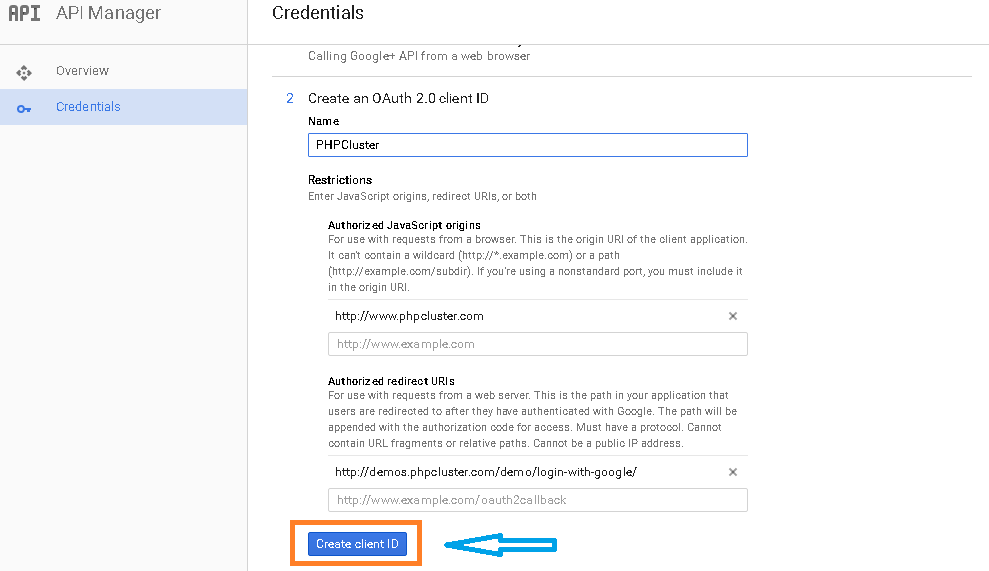
Now click on done button and by all the above steps we have created a project successfully in Google API Console. This part has finished. Now select project from top right corner and click on it and then click on Project Name to get client ID and Secret Key.
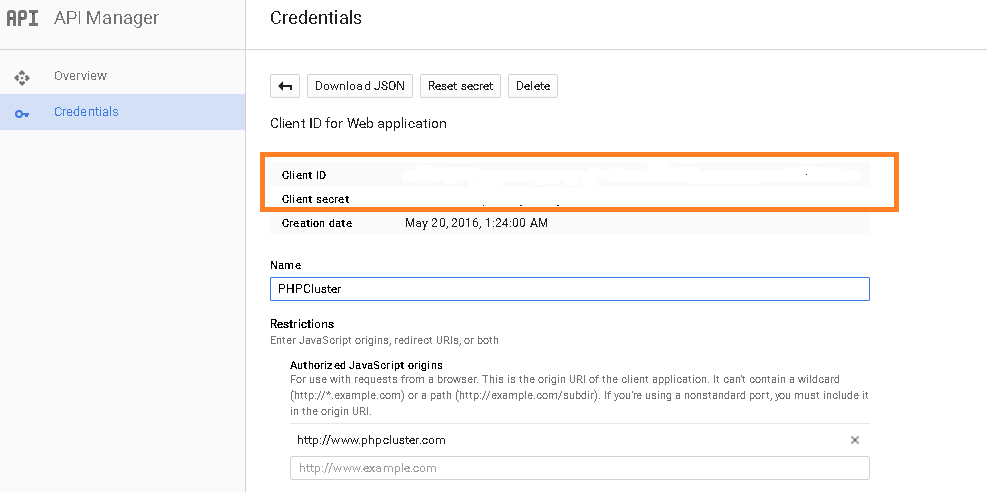
As we have got all required credential from above steps. Now e will see PHP snippet to get authenticate.
Step 3:
First of all to get authenticate we must have Google OAuth Library. We can get from Github repository and upload to project folder to get included in our project webpage.
Step 4:
Here we have created three PHP file and MySQL table to store record of authenticated users .
Config.php
Index.php
Logout.php
Mysql Table name = users
MySQLTable : users
Create the table to store information of user logged in with Google. Run the given below code in SQL query to create a table.
CREATE TABLE IF NOT EXISTS `users` ( `id` int(25) NOT NULL AUTO_INCREMENT, `name` varchar(100) NOT NULL, `email` varchar(100) NOT NULL, `created` timestamp NOT NULL DEFAULT CURRENT_TIMESTAMP ON UPDATE CURRENT_TIMESTAMP, PRIMARY KEY (`id`) ) ENGINE=MyISAM DEFAULT CHARSET=latin1 AUTO_INCREMENT=4 ;
config.php
This file is created to include Goole API library file and to get access token for authenticated user. Access token is stored in Session variable to get it access on index.php file.
<?php session_start(); //Google API Library includes include_once("src/Google_Client.php"); include_once("src/contrib/Google_Oauth2Service.php"); /*DB Config*/ mysql_connect('localhost','user','password'); mysql_select_db('DB Name'); //Client id, Client Secret, home url and redirect url $clientid= "Your Client ID "; $secret_key= "Your Secret Key"; $homeurl="Your Project Home URL"; $redirect_uri= "Redirect URL"; $api=new Google_Client(); $api->setApplicationName("Login to phpcluster"); $api->setClientId($clientid); $api->setClientSecret($secret_key); $api->setRedirectUri($redirect_uri); $google_oauth2 = new Google_Oauth2Service($api); //for session if(isset($_GET['code'])) { $api->authenticate($_GET['code']); $_SESSION['token']=$api->getAccessToken(); header('Location: ' . filter_var($redirect_uri, FILTER_SANITIZE_URL)); return; } if(isset($_SESSION['token'])) { $api->setAccessToken($_SESSION['token']); } //get login url when user not logged in if(!$api->getAccessToken()) { $loginurl= $api->createAuthUrl(); } else { //get user info when user logged in $userdetail = $google_oauth2->userinfo->get(); $_SESSION['email'] = $userdetail['email']; $_SESSION['profileurl']=$userdetail['link']; $_SESSION['name'] = $userdetail['name']; $_SESSION['profile_pic']= $userdetail['picture']; } ?>
index.php
This file is created to display user information which is stored in session variable after login.In this page we check for user is either user is new or not. If a user is new we store its info in database else we display a message of welcome back. See the snippet to get better.
<?php include ('config.php'); if($loginurl) { //user not logged in ?> <a href="<?php echo $loginurl; ?>" class="login">Login With Google</a> <?php } else{ echo "<h2>"."User Info:"."</h2>"."<br>"; echo "<b>"."Name:"."</b>".$_SESSION['name']."<br>"; echo "<b>"."email:"."</b>".$_SESSION['email'] ."<br>"; echo "<b>"."profileurl:"."</b>".$_SESSION['profileurl']."<br>"; echo "<b>"."profile picture:"."</b>".$_SESSION['profile_pic']."<br>"; $getemail = mysql_query("SELECT * FROM users WHERE `email`='".$_SESSION['email']."'"); if(mysql_num_rows($getemail)>0) { echo $_SESSION['newuser'] = "Welcome back".$_SESSION['name']; } else { if(!empty($_SESSION["email"])){ $gquery = mysql_query("INSERT INTO users SET name = '" .$_SESSION['name']. "',email='".$_SESSION["email"]."'") ; echo $_SESSION['newuser'] = "Thankyou for register"; } } ?> <img src="<?php echo $_SESSION['profile_pic']; ?>" height="50" width="50"> <a href="logout.php">logout</a> <?php } ?>
logout.php
This file is used to destroy the session and unset session variable. After logout user redirect to login page which is index page in this tutorial.
<?php include 'config.php'; //for logout unset session variable session_destroy(); unset($_SESSION['token']); $api->revokeToken(); header('Location: index.php'); ?>
That’s all. We successfully completed the script of login with Google.
Demo
[sociallocker]Download[/sociallocker]
If you loved this article 🙂 please share with your friends and colleagues 🙂 . If you have any query please write in comment.